16. Model Selection & Evaluation#
# Import some helper functions (please ignore this!)
from utils import *
Context: At this point, we have a general framework for developing probabilistic models, as well as one way of fitting them to data, MLE. We’ve then instantiated this framework to develop two types of predictive models—regression and classification. We further learned how to build in expressivity using tools from deep learning—namely, neural networks—into these models. Are we finally ready to apply these models to real-life tasks?
Unfortunately, there’s one key piece we’re still missing: so far, we’ve only used 1- and 2-dimensional input data and 1-dimensional output data. While in principle, we already have the tools to implement predictive models for higher dimensional data, we don’t yet have the tools to evaluate them. We’ve purposefully worked with lower dimensional data because it is easy to visualize, and therefore easy to qualitatively evaluate. But as data becomes higher dimensional, it’s much more difficult to get intuition using visualizations. As a result, we will have to rely on metrics.
Challenge: There are many ways of measuring model performance. Which metrics should we use? What are the pros and cons of each metric? We will answer these questions here. Even though our motivation for developing evaluation metrics is our inability to visualize high-dimensional data, we will in fact focus on low-dimensional data, again. This is because we need to gain intuition about each metric we introduce.
Outline:
What’s underfitting/overfitting? How do we prevent it?
Introduce log-likelihood
Introduce metrics specific to regression
Introduce metrics specific to classification
Data. You’ve started a collaboration with doctors from IHH’s Center for Rare Disorders. The doctors are interested in better understanding Antenna Inflammation, in which a being’s antennas sporadically inflame for weeks at a time. This causes them to malfunction and is quite painful. Because the disease is so rare, it’s been difficult to gather enough information to develop a treatment. Currently, the only known treatment is to expose the inflamed antennas to high-energy space beams. While these beams do not cure the disease, they do alleviate the pain. Doctors are interested in better understanding what beam intensity to use; they suspect that a low-intensity beam might not help much, but too high of an intensity might also contribute to pain. They have collected data for you to analyze. Let’s take a look:
# Import a bunch of libraries we'll be using below
import pandas as pd
import matplotlib.pylab as plt
import jax.numpy as jnp
import jax.random as jrandom
from cs349 import *
# Load the data into a pandas dataframe
csv_fname = 'data/IHH-CRD-train.csv'
data = pd.read_csv(csv_fname, index_col='Patient ID')
# Print a random sample of patients, just to see what's in the data
data.sample(15, random_state=0)
Intensity | Comfort | |
---|---|---|
Patient ID | ||
45 | 0.308006 | 0.977233 |
59 | 0.502982 | 1.359451 |
7 | 0.125683 | 0.405900 |
50 | 0.520344 | 1.185005 |
92 | 0.404359 | 0.955264 |
27 | 0.471006 | 1.138226 |
131 | 0.767512 | 1.025494 |
137 | 0.534193 | 0.828949 |
122 | 0.661706 | 1.179636 |
8 | 0.183754 | 0.742592 |
111 | 0.378024 | 0.670647 |
16 | 0.416132 | 0.664669 |
63 | 0.239045 | 0.871169 |
76 | 0.449077 | 0.933577 |
123 | 0.216425 | 0.722563 |
Models. In addition to the data the doctors have collected, they’ve gone ahead and fit some models to the data. They haven’t told you much about the modeling choices they made, but still want your help selecting which model best to use. Let’s load up the models:
models = load_all_regression_models_of_comfort_vs_intensity()
print('Number of models loaded:', len(models))
Number of models loaded: 6
We’ve created a helper function for you to visualize all of the models. Let’s have a look:
plot_all_regression_models_of_comfort_vs_intensity(data, models)
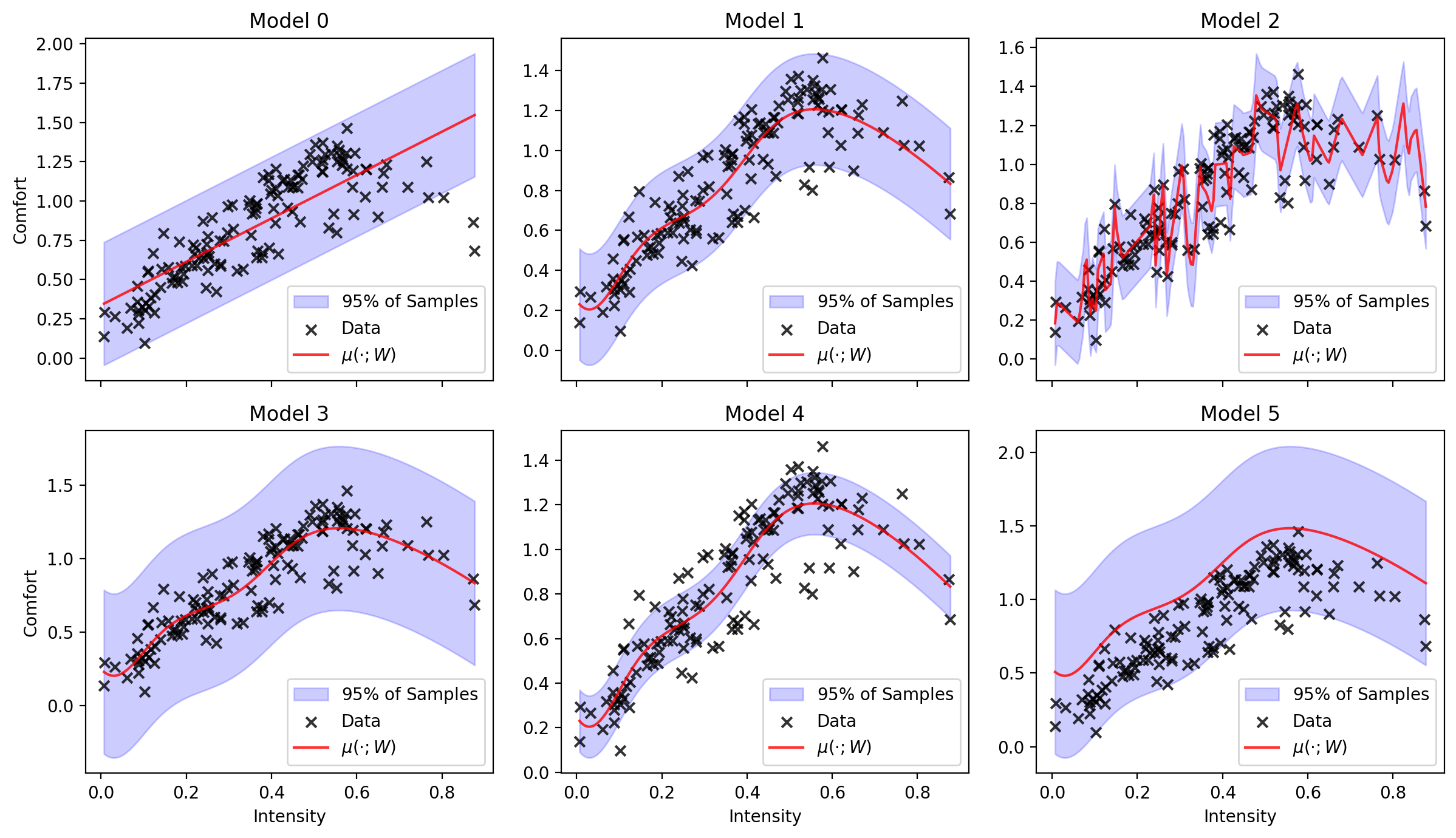
16.1. Preventing Over- and Under-fitting#
Decomposition into Signal vs. Noise. In probabilistic models, we often term one part of the model the “trend” or the “signal,” and we term the rest “noise” or “observation error.” For example, recall that in regression, our trend is captured by a function of the inputs,
Overfitting: When the “signal” part of the model memorizes the “noise” part, and as a result, interpolates poorly. By interpolation, we mean, how well the model fits data points near our training data.
Underfitting: When a model doesn’t capture enough of the “signal” in the data (assuming it’s noise), and as a result, interpolates poorly.
Let’s illustrate what overfitting and underfitting each look like, in both classification and regression:
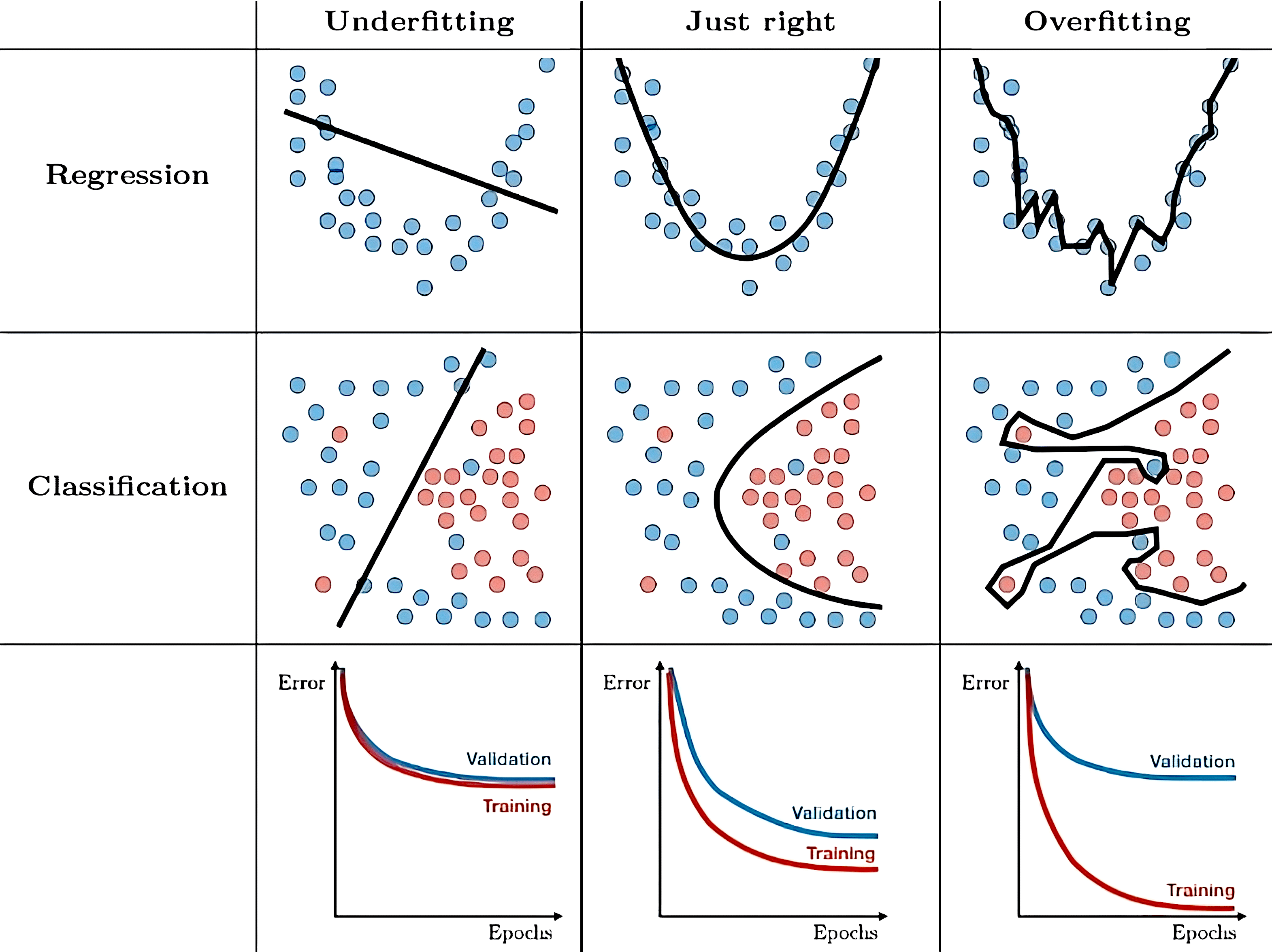
Fig. 16.1 Illustration of overfitting and underfitting, adapted from this image.#
Preventing Inappropriate Decomposition into Signal vs. Noise. How can we tell if a model overfit or underfit? Well, looking at the definition above, we said a model over/under-fitts if it interpolates poorly. This means that, to determine if a model over/under-fit, we should check its fit on data it has not seen yet—specifically, on data that’s similar to our training data.
But where can we get such extra data points? Oftentimes, it’s hard to collect additional data. For example, at the IHH, data collection is expensive; it’s funded by large government grants and requires approval of an Institutional Review Board (IRB) to ensure data collection and analysis is ethical. As a result, we often cannot collect additional data. Instead, however, we can split our data into parts—one part for training and one part for validating the model’s interpolation. Specifically, after fitting the model on the training data, we compare the model’s fit on the training data with its fit on the validation data:
If the fit on the training data is better than that on the validation data, we overfit.
If the fit on neither the training data nor the validation data is good, we underfit.
If the fit on both the training and validation data is comparably good, we fit just right!
The bottom row of Fig. 16.1 above shows the loss function evaluated on both the training data and the validation set over iterations (or “epochs”) of gradient descent.
Reporting Model Performance. In practice, we often aren’t just interested in the fitted model—we also want to report some metric to quantify how well it fits. To accomodate this need, we split the data into three parts: a training set, a validation set, and a test set.
We fit the model to the training data.
We use the validation set to determine over/under-fitting (by comparing the fit on the validation vs. training data, as described above).
Finally, we report the model’s performance on the test set.
Often, we use
But which metric should we use? That’s a million-dollar question! We will next present several common metrics for evaluating model performance numerically. As you will see, model performance is multi-faceted—there’s more to consider than just how well the model fits. For example, is the model fair? and will the model be understandable to a human? If we have multiple factors to consider, how can we possibly design a metric that gives us a single ranking of models from best to worst? The answer is: we can’t. We will have to use multiple metrics to evaluate our models. At times, these metrics will present us with contradictory information, and we will have to determine what to do.
16.2. Log-Likelihood#
A General Purpose Metric. Since so far, we fit our models by finding parameters that maximize the data log-likelihood (MLE). Can we also use log-likelihood as an evaluation metric? If we trust this metric for fitting our model, surely we should trust it for evaluation as well. And what’s nice about log-likelihood is that, unlike some of the other metrics we’ll introduce next, it’s not model-specific. We can evaluate the log-likelihood of any probabilistic model.
Log-Likelihood as an Evaluation Metric. Recall that the data log-likelihood is,
where
Exercise: Getting Intuition for Log-Likelihood
Part 1: Looking at the plots of the models above, rank them from what you’d consider best to worst. How did you arrive at this ranking? Please relate your answer to the broader context of the problem.
Part 2: For each model, evaluate the average log-likelihood on the training, validation, and test set. Do this by,
Loading in the list of models (using the function we provided above). Each model in the list has the following signature:
def model(N, x, y=None): # Expects x to be of shape (N, 1) # Expects y to be of shape (N, 1) pass # already implemented
Here,
N
is the number of points,x
are the inputs, andy
are the outputs.Loading the training, validation, and test data sets from
data/IHH-CRD-train.csv
,data/IHH-CRD-val.csv
, anddata/IHH-CRD-test.csv
.Finally, compute the average log-likelihood. Please use the helper function we created,
cs349_joint_data_log_likelihood(model, *args, **kwargs)
, where*args, **kwargs
capture all arguments your model takes in:N, x, y=None
. Note that our helper function computes the joint data log-likelihood, not the average log-likelihood.
Part 3: Rank the models using log-likelihood on the training set and report log-likelihood test set. Did this method succeed in ranking the best-fitting model on top? Next, rank the models using validation set and report the test log-likelihood. Did this method successfully rank the best-fitting model on top?
Part 4: How did the ranking you got from using the validation set compare with your initial ranking from part (1)? What does log-likelihood penalize models for?
Human-meaningful Notions of Model-fit. So log-likelihood seems to work well when comparing models; given a collection of trained models, we can use log-likelihood on the validation set to select a model that is likely to fit the test set well. However, suppose we’re given a single model—how do we know if this model is good? For example, if our model’s log-likelihood is
16.3. Metrics for Regression#
Mean Squared Error (MSE). Since log-likelihood is hard to interpret on its own, we will now introduce another metric, MSE, specific to regression. This metric can be used together with log-likelihood to paint a clearer picture of model fit. This is, of course, not the only evaluation metric for regression, but it’s a common one, so we’ll focus on it here. For 1-dimensional output data, the MSE is defined as follows:
where
But for a probabilistic model, what is the model’s “prediction”? In a probabilistic regression model, we have a distribution of outputs given inputs,
Exercise: Implement and Use MSE
Part 1: Write a function to compute the MSE. The models are set up such that calling cs349_sample_generative_process
will return a Python dictionary with key mu
, containing
Part 2: For each model, evaluate the MSE on the training, validation, and test set.
Part 3: Rank the models using validation MSE and report the test MSE. Does this ranking disagree with the log-likelihood ranking? If so, where? Hint: look at the observation noise.
Part 4: Are there any other factors we should have considered in our evaluation of the models? Let’s load the training, validation, and test data sets from data/IHH-CRD-train-augmented.csv
, data/IHH-CRD-val-augmented.csv
, and data/IHH-CRD-test-augmented.csv
. These data sets are the same as before, just with a previously omitted feature: race. As it turns out, the intervention (the space beam), was only tested on one of these races and not the other. Create a scatter plot of the training data using color to distinguish between the two races.
What’s different about the trend for the two races? What’s the same?
How do you think this will affect the model’s predictive performance on each of the two races? Why?
Part 5: Re-compute the log-likelihood of each model on each of these data sets, this time evaluating it separately for each race. For which race does the model perform better on? Why?
16.4. Metrics for Classification#
Accuracy. Since log-likelihood is hard to interpret on its own, we will now introduce another metric, accuracy, that’s specific to classification. Simply put, accuracy is the fraction of predictions the model got correct:
where, as before,
Just like before, a probabilistic model does not make “predictions;” it models a full distribution over outputs given inputs. We, again, have to choose how to summarize this distribution using a single value. Remember that in our classification model,
Exercise: The Shortcomings of Accuracy
Context. Since the disease is incredibly rare, occurring only in
Challenge. Even though their model is
Part 1: You decided to inspect the parameters of the model. In the model,
where
where the
Looking at the parameters,
Part 2: How can such a model achieve such high accuracy?
Part 3: Suppose the IHH were to deploy this model as a screening tool for beings coming into the IHH’s emergency room. The model would be used to identify beings at high risk of Antenna Infection for early treatment. What problems may arise?
Confusion Matrices. Although accuracy is easy to evaluate and understand, it has some major shortcomings, as you’ve shown in the exercise above. Confusion matrices aim to address this issue by looking at the ways in which the model makes mistakes when it’s incorrect. Why are they called “confusion” matrices? Maybe to alleviate our confusion? Unclear. Anyways, we define a confusion matrix as follows:
Predicted: Disease |
Predicted: No Disease |
|
---|---|---|
Actual: Disease |
True Positives: The number of patients with the disease who were correctly classified as having the disease. |
False Negatives: The number of patients with the disease who were incorrectly classified as not having the disease. |
Actual: No Disease |
False Positives: The number of patients without the disease who were incorrectly classified as having the disease. |
True Negatives: The number of patients without the disease who were correctly classified as having no disease. |
As you can see, a confusion matrix presents a breakdown of the model’s correct and incorrect predictions.
Exercise: Understanding Confusion Matrices
Part 1: Write down the confusion matrix for the classifier presented to you by the doctors in the previous problems (in terms of the number of patients in the data,
Part 2: To try to fix the problem, the doctors trained two more classifiers on their data set. As per your suggestion, they are now computing the confusion matrices for both (instead of looking at accuracy alone):
Model 1:
Model 2:
For the screening tool described in the previous problem, which model would you prefer? Why?
Part 3: Make up a scenario in which you would prefer the model you didn’t choose? What differentiates your scenario from the one about the screening tool?